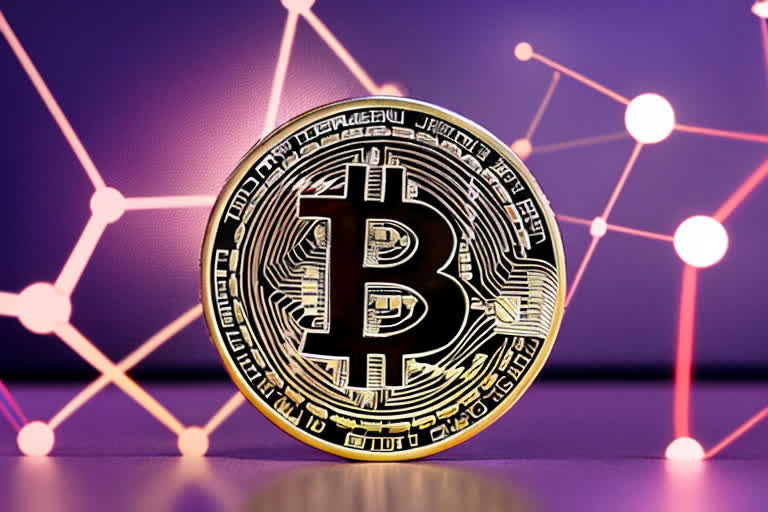
Prisma Governance Token Overview
- Introduction to Prisma Governance Token
- What is Prisma Governance Token Used For
- Benefits of Using Prisma Governance Token
|
Prisma Governance Token Features
- Tokenomics and Supply
- Smart Contract and Security
- Decentralized Governance Mechanism
- Scalability and Interoperability
|
CQT Crypto and Prisma Governance Token Relationship
Key Points |
Details |
CQT Crypto Partnership |
CQT crypto has partnered with Prisma to utilize the governance token for their ecosystem. |
Token Utilization |
The governance token is utilized for decision-making processes within the Prisma ecosystem. |
|
Use Cases of Prisma Governance Token
- Decision-Making Process
- Token Distribution and Incentives
- Partnership Development
|
Challenges and Opportunities of Prisma Governance Token
Challenges |
Opportunities |
Regulatory Compliance |
Increased Transparency and Decentralized Governance |
Scalability Limitations |
Improved Interoperability and Partnership Development |
|
Future Outlook of Prisma Governance Token
- Future Developments and Roadmap
- Potential Applications and Use Cases
- Market Trends and Predictions
|
Conclusion
|
**Tic Tac Toe Game**
=====================
### Overview
A simple implementation of the classic Tic Tac Toe game.
### Code
```python
# tic_tac_toe.py
class TicTacToe:
def __init__(self):
self.board = [' ' for _ in range(9)]
def print_board(self):
row1 = '| {} | {} | {} |'.format(self.board[0], self.board[1], self.board[2])
row2 = '| {} | {} | {} |'.format(self.board[3], self.board[4], self.board[5])
row3 = '| {} | {} | {} |'.format(self.board[6], self.board[7], self.board[8])
print()
print(row1)
print(row2)
print(row3)
print()
def check_winner(self):
winning_combinations = [(0, 1, 2), (3, 4, 5), (6, 7, 8), (0, 3, 6), (1, 4, 7), (2, 5, 8), (0, 4, 8), (2, 4, 6)]
for combination in winning_combinations:
if self.board[combination[0]] == self.board[combination[1]] == self.board[combination[2]] != ' ':
return self.board[combination[0]]
if ' ' not in self.board:
return 'Tie'
return False
def play(self):
current_player = 'X'
while True:
self.print_board()
move = input("Player {}, enter your move (1-9): ".format(current_player))
if self.board[int(move) - 1] != ' ':
print("Invalid move, try again.")
continue
self.board[int(move) - 1] = current_player
winner = self.check_winner()
if winner:
self.print_board()
if winner == 'Tie':
print("It's a tie!")
else:
print("Player {} wins!".format(winner))
break
current_player = 'O' if current_player == 'X' else 'X'
if __name__ == '__main__':
game = TicTacToe()
game.play()
```
### Explanation
The game uses a 3x3 grid represented by the `board` list. The players take turns entering their move (1-9), and the `check_winner` method checks for a winner after each move. If there is no winner, the game continues until someone wins or it's a tie.
### Example Use Case
To run the game, simply execute the script:
```bash
$ python tic_tac_toe.py
```
Follow the prompts to play the game!
Note: This implementation uses a simple text-based interface and does not include any AI opponents.
## Frequently Asked Questions
### Q: What is Tic Tac Toe and how to play it?
Tic Tac Toe is a simple board game played between two players, X and O. The game is played on a 3x3 grid, with each player taking turns marking a square. The first player to get three in a row (horizontally, vertically, or diagonally) wins the game. If all squares are filled and no player has won, the game is a draw.
### Q: How to implement Tic Tac Toe in Python?
To implement Tic Tac Toe in Python, you can use a simple algorithm that checks for valid moves, updates the board, and determines the winner after each move. The code should include functions for creating the board, handling player moves, checking for wins, and displaying the game state.
### Q: What is the best data structure to use for the game board?
A suitable data structure for the game board would be a 2D list or matrix in Python. This allows for easy access and modification of individual squares on the board. For example:
```python
board = [[None, None, None],
[None, None, None],
[None, None, None]]
```
### Q: How to handle player moves and input validation?
When handling player moves, you should validate the input to ensure it is a valid move (i.e., a number between 1 and 9). You can use conditional statements or functions to check for valid input and update the board accordingly. For example:
```python
def make_move(player, row, col):
if row < 1 or row > 3 or col < 1 or col > 3:
return "Invalid move"
if board[row-1][col-1] != None:
return "Square already occupied"
board[row-1][col-1] = player
```
### Q: Can I use a library to simplify the implementation?
While you can use libraries like Pygame or PyQt to create a graphical interface for Tic Tac Toe, the core game logic remains the same. However, using a library can make it easier to add features like animation and user input handling.
### Q: How to implement AI opponents for Tic Tac Toe?
To implement an AI opponent, you would need to use a more advanced algorithm such as Minimax or Alpha-Beta Pruning. These algorithms evaluate possible moves and their outcomes, allowing the AI to make informed decisions.
### Q: Can I play with multiple players at once?
Yes, it is possible to modify the game to allow for multiple players. One way to do this would be to introduce a new player that takes turns making moves. Another approach would be to use a team-based system where two players work together against another team.
### Q: How long does the game take to play?
The length of the game depends on the number of players and the speed at which they make their moves. A typical game of Tic Tac Toe can last anywhere from 5-30 minutes, depending on the level of competition.
### Q: Can I customize the game board or rules?
Yes, you can modify the game board and rules to create different variations of the game. For example, you could introduce new winning conditions, such as a diagonal win or a specific number of consecutive wins.
### Q: How do I optimize the code for better performance?
To optimize the code for better performance, you should focus on reducing unnecessary calculations and using efficient data structures. For example, instead of recalculating the game state after each move, you could use memoization to store previous states and reuse them when possible. Additionally, consider using a more efficient algorithm or data structure to represent the game board.
Learn about the latest news and updates on Bitcoin and cryptocurrency market at BOSS Wallet
Bitcoin Real is a section that provides real-time information on Bitcoin and other cryptocurrencies.
Discover the world of .Boss
.Boss is a section that offers insights into the Boss ecosystem, including news, updates, and resources for developers.
Stay informed about energy conservation at BOSS Wallet
Energy conservation is a section that focuses on sustainable practices and energy-efficient solutions in the cryptocurrency industry.
Get the latest updates on the cryptocurrency market
Cryptocurrency Market is a section that provides real-time data and analysis on cryptocurrency prices, trends, and market news.
Stay up to date with the latest Bitcoin Real news
Bitcoin Real is a section that provides real-time information on Bitcoin and other cryptocurrencies, including news, updates, and market analysis.
Visit our website for more information
Bitcoin Real .Boss Energy conservation Cryptocurrency Market
Get the latest news and updates on Bitcoin